- MT5(C++)软件编程十大排序算法 - 冒泡排序算法 (bubble Sort)
- MT5(C++)软件编程十大排序算法 - 选择排序算法 (select Sort)
- MT5(C++)软件编程十大排序算法 - 插入排序算法 (insert Sort)
- MT5(C++)软件编程十大排序算法 - 快速排序算法 (quick Sort)
- MT5(C++)软件编程十大排序算法 - 希尔排序算法 (shell Sort)
- MT5(C++)软件编程十大排序算法 - 堆排序算法 (heap Sort)
- MT5(C++)软件编程十大排序算法 - 归并排序算法 (merge Sort)
- MT5(C++)软件编程十大排序算法 - 计数排序算法 (counting Sort)
- MT5(C++)软件编程十大排序算法 - 桶排序算法 (bucket Sort)
- MT5(C++)软件编程十大排序算法 - 基数排序算法 (radix Sort)
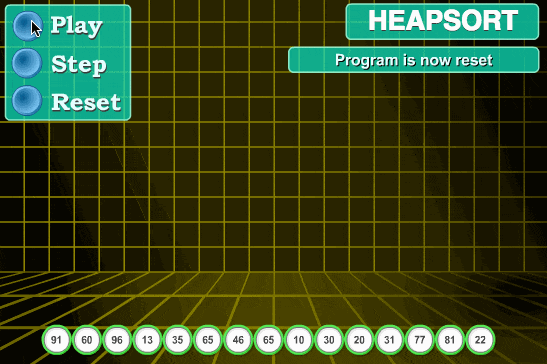
堆排序算法
以二分之一分递归分割数组
arr: 需要排序的数组
seq: 排序的方向, true 为升序, false为降序
template <typename T>
void heapSort(T &arr[],const bool seq = true)
{
int len = ArraySize(arr);
int start = len;
int end = len - 1;
while(start >= 0)
{
big_endian(arr,start,end,seq);
start -= 1;
}
while(end > 0)
{
swap(arr[0],arr[end]);
big_endian(arr,0,end -1,seq);
end -= 1;
}
}
template <typename T>
void big_endian(T &arr[], int start, int end, const bool seq = true)
{
int root = start;
int child = root * 2 + 1;
if(seq)
while(child <= end)
{
if(child + 1 <= end && arr[child] < arr[child + 1])
{
child += 1;
}
if(arr[root] < arr[child])
{
swap(arr[root],arr[child]);
root = child;
child = root * 2 + 1;
}
else break;
}
else
while(child <= end)
{
if(child + 1 <= end && arr[child] > arr[child + 1])
{
child += 1;
}
if(arr[root] > arr[child])
{
swap(arr[root],arr[child]);
root = child;
child = root * 2 + 1;
}
else break;
}
}